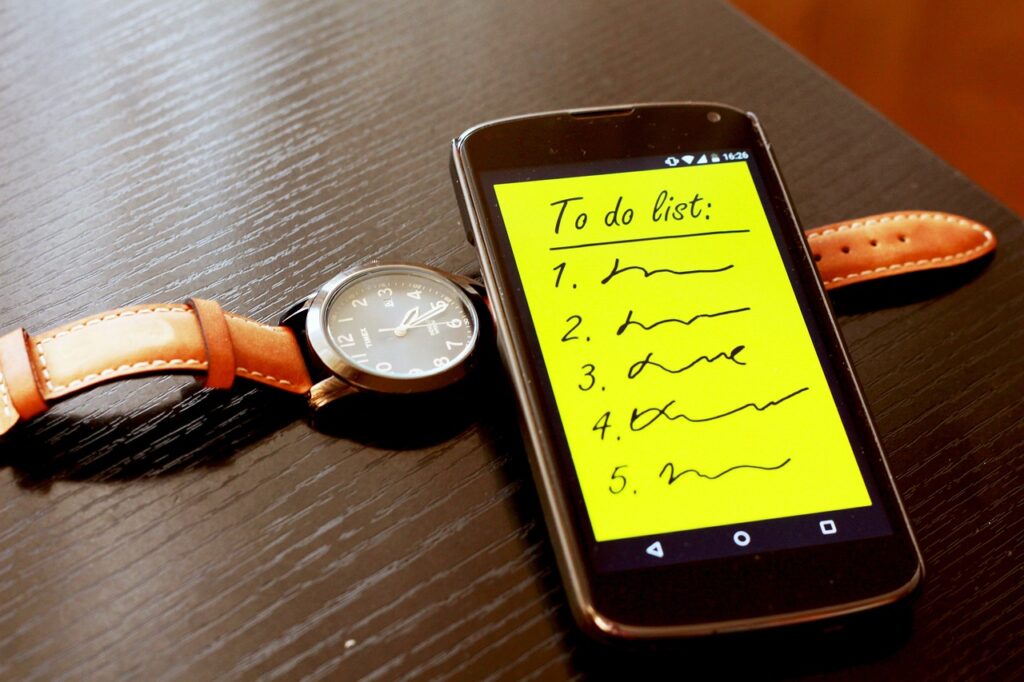
Souce code: https://github.com/fenelon01/TODO-APP
Code explanation here:
Event Listener for DOM Content Loaded
javascriptCopy codedocument.addEventListener('DOMContentLoaded', function() {
- Purpose: This event listener ensures that the JavaScript code only runs after the HTML document has been fully loaded and parsed. This is important because it ensures that all the elements we want to manipulate in the DOM are available.
2. Selecting DOM Elements
javascriptCopy codeconst taskInput = document.getElementById('task-input');
const taskDate = document.getElementById('task-date');
const taskTime = document.getElementById('task-time');
const taskList = document.getElementById('task-list');
const addTaskBtn = document.getElementById('add-task-btn');
- Purpose: These lines select specific HTML elements using their IDs so that we can interact with them. For example:
taskInput
is the text input where users type their task.taskDate
andtaskTime
are the input fields for selecting the due date and time of a task.taskList
is the unordered list (<ul>
) where tasks will be displayed.addTaskBtn
is the button that users click to add a new task.
3. Adding Event Listener for the Add Task Button
javascriptCopy codeaddTaskBtn.addEventListener('click', function() {
- Purpose: This adds a click event listener to the "Add Task" button. When the button is clicked, the function inside this event listener will run, which handles adding a new task to the list.
4. Creating a New Task
javascriptCopy code const taskText = taskInput.value;
const taskDeadline = `${taskDate.value} ${taskTime.value}`;
const isPriority = document.getElementById('priority').checked;
if (taskText !== '') {
const li = document.createElement('li');
li.innerHTML = `
<span class="task-text">${taskText}</span>
<span class="countdown"></span>
<span class="task-deadline">${taskDeadline}</span>
<input type="checkbox" class="done-checkmark">
<button class="delete-btn">Delete</button>
`;
if (isPriority) {
li.classList.add('priority');
}
taskList.appendChild(li);
taskInput.value = '';
taskDate.value = '';
taskTime.value = '';
updateCountdowns();
}
- Explanation:
taskText
: Retrieves the value of the task input field.taskDeadline
: Combines the selected date and time to create a deadline for the task.isPriority
: Checks if the priority checkbox is selected.- If condition: Ensures that the task text isn’t empty before creating a new task.
li
element creation: A new list item (<li>
) is created, and its inner HTML is set to include the task text, deadline, a checkbox for marking the task as done, and a delete button.- Priority Check: If the task is marked as a priority, the
priority
class is added to the list item. - Clearing Input Fields: After the task is added, the input fields are cleared.
- updateCountdowns(): This function updates the countdown timers for all tasks.
5. Delete Task Functionality
javascriptCopy codetaskList.addEventListener('click', function(e) {
if (e.target.classList.contains('delete-btn')) {
e.target.parentElement.remove();
}
});
- Purpose: This listens for a click event anywhere within the
taskList
(where all tasks are displayed). If the clicked element has the classdelete-btn
(i.e., the delete button for a task), the task’s parent element (the<li>
containing the task) is removed from the DOM.
6. Mark Task as Done
javascriptCopy codetaskList.addEventListener('change', function(e) {
if (e.target.classList.contains('done-checkmark')) {
const listItem = e.target.parentElement;
listItem.classList.toggle('completed');
}
});
- Purpose: This listens for changes (like checking a checkbox) in the
taskList
. If the change occurs on an element with the classdone-checkmark
(i.e., the task’s checkbox), it toggles thecompleted
class on the task’s<li>
element, which will visually strike through the task.
7. Updating Countdown Timers
javascriptCopy codefunction updateCountdowns() {
const tasks = taskList.querySelectorAll('li');
tasks.forEach(task => {
const deadline = new Date(task.querySelector('.task-deadline').textContent);
const now = new Date();
const diff = deadline - now;
if (diff > 0) {
const hours = Math.floor((diff % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
const minutes = Math.floor((diff % (1000 * 60 * 60)) / (1000 * 60));
task.querySelector('.countdown').textContent = `${hours}h ${minutes}m left`;
} else {
task.querySelector('.countdown').textContent = 'Missed!';
}
});
}
setInterval(updateCountdowns, 60000);
- Purpose: This function calculates the time left until each task’s deadline and updates the countdown timers displayed next to each task.
- Deadline Calculation: It parses the deadline from the task’s HTML and calculates the difference between the current time (
now
) and the task’s deadline. - Time Remaining: If the difference is positive (meaning the deadline hasn’t passed), the hours and minutes remaining are calculated and displayed. If the deadline has passed, the text "Missed!" is displayed.
- setInterval: This runs the
updateCountdowns
function every minute to keep the countdowns up to date.
- Deadline Calculation: It parses the deadline from the task’s HTML and calculates the difference between the current time (
8. Closing the DOMContentLoaded Event Listener
javascriptCopy code});
- Purpose: This closes the initial
DOMContentLoaded
event listener that encapsulates all the functionality, ensuring that the script runs only after the document is ready.
Summary
- DOM Manipulation: The script selects and manipulates DOM elements to create, delete, and manage tasks.
- Event Listeners: It uses event listeners to handle user interactions, such as adding tasks, marking them as done, and deleting them.
- Countdown Timers: It includes logic for updating countdown timers to keep users aware of approaching deadlines.